안녕하세요. Point-Checks입니다.
이번 포스팅에서는 AI 기반 비트코인 자동 매매 프로젝트 – Day 2를 진행하겠습니다. 오늘은 비트코인 관련 최신 뉴스를 가져오는 방법과 인기 뉴스(Rising, Hot)를 수집하는 방법을 다룹니다. AI가 분석할 데이터를 확보하는 중요한 과정입니다.
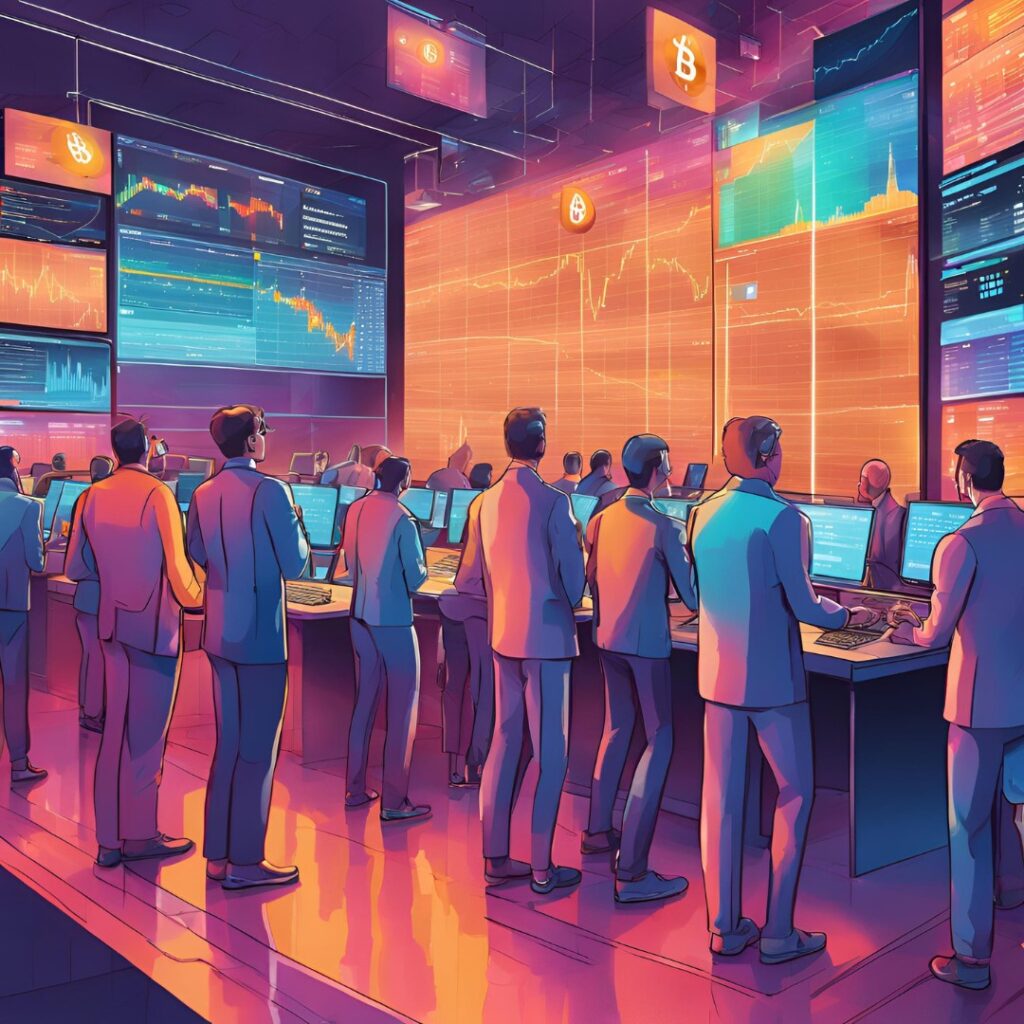
비트코인 뉴스 데이터 가져오기
1. CryptoPanic API 및 라이브러리 설치
비트코인 관련 뉴스를 수집하기 위해 requests
라이브러리를 사용합니다.
📌 requirements.txt
requests
json
위 라이브러리를 설치하려면 다음 명령어를 실행하세요.
pip install -r requirements.txt
2. 최근 3시간 내 비트코인 뉴스 가져오기
아래 recent.py
코드를 실행하면 CryptoPanic API를 통해 비트코인 관련 최신 뉴스 데이터를 가져오고 출력합니다.
📌 recent.py
import requests
import json
import datetime
def get_bitcoin_news():
url = "https://cryptopanic.com/api/v1/posts/"
params = {
"auth_token": "your API token", # your API token
"currencies": "BTC" # 비트코인(BTC) 관련 모든 포스트 요청
}
response = requests.get(url, params=params)
if response.status_code == 200:
return response.json()
else:
print("오류 발생:", response.status_code)
return None
def format_time_delta(published_at, now):
"""
Returns a string representing the time elapsed between 'now' and 'published_at'
in the format "X hours Y minutes ago".
"""
delta = now - published_at
total_seconds = int(delta.total_seconds())
hours = total_seconds // 3600
minutes = (total_seconds % 3600) // 60
return f"{hours} hours {minutes} minutes ago"
def summarize_news(news_data):
"""
최근 3시간 내의 모든 포스트(뉴스, 트윗 등) 항목에서 제목과 함께
'X hours Y minutes ago' 형식의 시간을 출력합니다.
- 포스트 건수
- 제목 및 경과 시간 리스트
"""
now = datetime.datetime.now(datetime.timezone.utc)
news_items = news_data.get("results", [])
recent_posts = []
for item in news_items:
published_str = item.get("published_at")
if published_str:
# ISO 형식 날짜 문자열을 UTC offset-aware datetime 객체로 변환
published_at = datetime.datetime.fromisoformat(published_str.replace("Z", "+00:00"))
if (now - published_at).total_seconds() <= 3 * 3600: # 최근 3시간 내
title = item.get("title", "")
if title:
time_ago = format_time_delta(published_at, now)
recent_posts.append({
"title": title,
"time_ago": time_ago
})
news_count = len(recent_posts)
if news_count == 0:
return None
summary = {
"news_count": news_count,
"posts": recent_posts
}
return summary
if __name__ == "__main__":
news_data = get_bitcoin_news()
if news_data:
summary = summarize_news(news_data)
if summary:
print("최근 3시간 내 포스트 제목 및 경과 시간 요약:")
print(json.dumps(summary, indent=4, ensure_ascii=False))
else:
print("최근 3시간 내의 포스트가 없습니다.")
3. Rising & Hot 뉴스 가져오기
아래 rising.py
와 hot.py
코드를 실행하면 인기 상승 중(Rising) 및 Hot 뉴스 데이터를 가져올 수 있습니다.
📌 rising.py
import requests
import json
import datetime
def get_rising_bitcoin_news():
url = "https://cryptopanic.com/api/v1/posts/"
params = {
"auth_token": "your API token", # your API token
"currencies": "BTC", # Request BTC-related posts
"filter": "rising" # Use the "rising" filter
}
response = requests.get(url, params=params)
if response.status_code == 200:
return response.json()
else:
print("Error occurred:", response.status_code)
return None
def format_time_delta(published_at, now):
"""
Returns a string representing the time elapsed between 'now' and 'published_at'
in the format "X hours Y minutes ago".
"""
delta = now - published_at
total_seconds = int(delta.total_seconds())
hours = total_seconds // 3600
minutes = (total_seconds % 3600) // 60
return f"{hours} hours {minutes} minutes ago"
def summarize_rising_news(news_data):
"""
Processes the rising posts (without a time constraint) and returns a summary
with up to 10 items. Each item includes the title and how long ago it was published.
"""
now = datetime.datetime.now(datetime.timezone.utc)
news_items = news_data.get("results", [])
summary_list = []
for item in news_items:
published_str = item.get("published_at")
if published_str:
# Convert the ISO date string to a UTC offset-aware datetime object
published_at = datetime.datetime.fromisoformat(published_str.replace("Z", "+00:00"))
title = item.get("title", "")
time_ago = format_time_delta(published_at, now)
summary_list.append({
"title": title,
"time_ago": time_ago
})
# Stop once we have 10 items
if len(summary_list) >= 10:
break
summary = {
"news_count": len(summary_list),
"posts": summary_list
}
return summary
if __name__ == "__main__":
news_data = get_rising_bitcoin_news()
if news_data:
summary = summarize_rising_news(news_data)
if summary and summary["news_count"] > 0:
print("Rising posts summary:")
print(json.dumps(summary, indent=4, ensure_ascii=False))
else:
print("No rising posts found.")
📌 hot.py
import requests
import json
import datetime
def get_hot_bitcoin_news():
url = "https://cryptopanic.com/api/v1/posts/"
params = {
"auth_token": "your API token", # your API token
"currencies": "BTC", # Request BTC-related posts
"filter": "hot" # Use the "hot" filter
}
response = requests.get(url, params=params)
if response.status_code == 200:
return response.json()
else:
print("Error occurred:", response.status_code)
return None
def format_time_delta(published_at, now):
"""
Returns a string representing the time elapsed between 'now' and 'published_at'
in the format "X hours Y minutes ago".
"""
delta = now - published_at
total_seconds = int(delta.total_seconds())
hours = total_seconds // 3600
minutes = (total_seconds % 3600) // 60
return f"{hours} hours {minutes} minutes ago"
def summarize_hot_news(news_data):
"""
Processes the hot posts (without a time constraint) and returns a summary
with up to 10 items. Each item includes the title and how long ago it was published.
"""
now = datetime.datetime.now(datetime.timezone.utc)
news_items = news_data.get("results", [])
summary_list = []
for item in news_items:
published_str = item.get("published_at")
if published_str:
# Convert the ISO date string to a UTC offset-aware datetime object
published_at = datetime.datetime.fromisoformat(published_str.replace("Z", "+00:00"))
title = item.get("title", "")
time_ago = format_time_delta(published_at, now)
summary_list.append({
"title": title,
"time_ago": time_ago
})
# Stop once we have 10 items
if len(summary_list) >= 10:
break
summary = {
"news_count": len(summary_list),
"posts": summary_list
}
return summary
if __name__ == "__main__":
news_data = get_hot_bitcoin_news()
if news_data:
summary = summarize_hot_news(news_data)
if summary and summary["news_count"] > 0:
print("Hot posts summary:")
print(json.dumps(summary, indent=4, ensure_ascii=False))
else:
print("No hot posts found.")
📌 결과 예시
{
"news_count": 10,
"posts": [
{
"title": "Bitcoin’s Next Rally Around the Corner as Stablecoin Liquidity Expands: CryptoQuant",
"time_ago": "1 hours 8 minutes ago"
},
{
"title": "European Central Bank president: Bitcoin lacks reserve potential",
"time_ago": "8 hours 35 minutes ago"
},
{
"title": "Texas to Prioritize Bitcoin Reserve in 2025",
"time_ago": "23 hours 30 minutes ago"
},
{
"title": "Robert Kiyosaki Ditches Gold and Silver, Goes All-In on Bitcoin",
"time_ago": "26 hours 6 minutes ago"
},
{
"title": "Tether Brings USDT to Bitcoin’s Lightning Network for Faster, Cheaper Payments",
"time_ago": "27 hours 49 minutes ago"
},
{
"title": "European Central Bank President Dismisses BTC as Viable Reserve Asset",
"time_ago": "30 hours 26 minutes ago"
},
{
"title": "Trump Media Announces $250M Crypto and ETF Investment Strategy",
"time_ago": "36 hours 35 minutes ago"
},
{
"title": "US SEC approves Bitwise’s combined Bitcoin and Ethereum ETF",
"time_ago": "37 hours 2 minutes ago"
},
{
"title": "Trump Launches Official Watches—Pay with Bitcoin or $TRUMP",
"time_ago": "46 hours 8 minutes ago"
},
{
"title": "Indiana Moves to Allow Bitcoin in Pension Funds as States Eye Crypto Reserves",
"time_ago": "47 hours 6 minutes ago"
}
]
}
🔍 마무리
이번 포스팅에서는 CryptoPanic API를 사용하여 비트코인 관련 최신 뉴스와 인기 뉴스(Rising, Hot)를 가져오는 방법을 살펴보았습니다. 이제 우리는 AI에게 제공할 중요한 정보 데이터를 확보하는 과정을 완료했습니다.
다음 포스팅에서는 AI가 이 데이터를 분석하여 투자 결정을 내리는 방법을 살펴보겠습니다! 🚀